Word Search (Leetcode 79) - Top Coding Interview Question
Given an m x n
grid of characters board
and a string word
, return true
if word
exists in the grid.
The word can be constructed from letters of sequentially adjacent cells, where adjacent cells are horizontally or vertically neighboring. The same letter cell may not be used more than once.
Example 1:
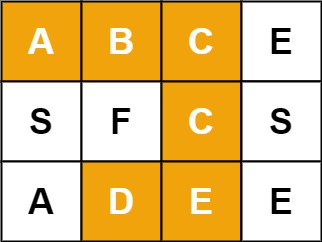
Input: board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "ABCCED" Output: true
Example 2:
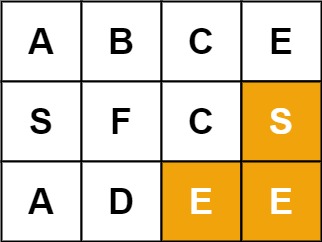
Input: board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "SEE" Output: true
Example 3:
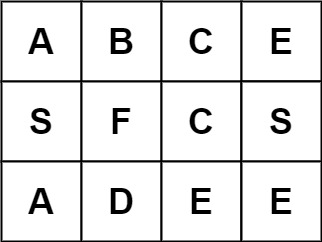
Input: board = [["A","B","C","E"],["S","F","C","S"],["A","D","E","E"]], word = "ABCB" Output: false
Constraints:
m == board.length
n = board[i].length
1 <= m, n <= 6
1 <= word.length <= 15
board
andword
consists of only lowercase and uppercase English letters.
Follow up: Could you use search pruning to make your solution faster with a larger board
?
Solution:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
/**
* Time Complexity: O(N * 3 ^ L) : N is the number of cells.
* Space Complexity: O(m * n) for a mxn board.
**/
class Solution {
public boolean exist(char[][] board, String word) {
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board[0].length; j++) {
backtrack(board, word, i, j, new boolean[board.length][board[0].length]);
if (result) {
return result;
}
}
}
return false;
}
boolean result = false;
void backtrack(char[][] board, String word, int i, int j, boolean[][] visited) {
if (word.length() == 0) {
result = true;
return;
}
if (i < 0 || i >= board.length || j < 0 || j >= board[0].length
|| visited[i][j] || word.charAt(0) != board[i][j]) {
return;
}
visited[i][j] = true;
backtrack(board, word.substring(1), i, j + 1, visited);
backtrack(board, word.substring(1), i, j - 1, visited);
backtrack(board, word.substring(1), i + 1, j, visited);
backtrack(board, word.substring(1), i - 1, j, visited);
visited[i][j] = false;
}
}