3 simple ways to get the first character of a string in Golang
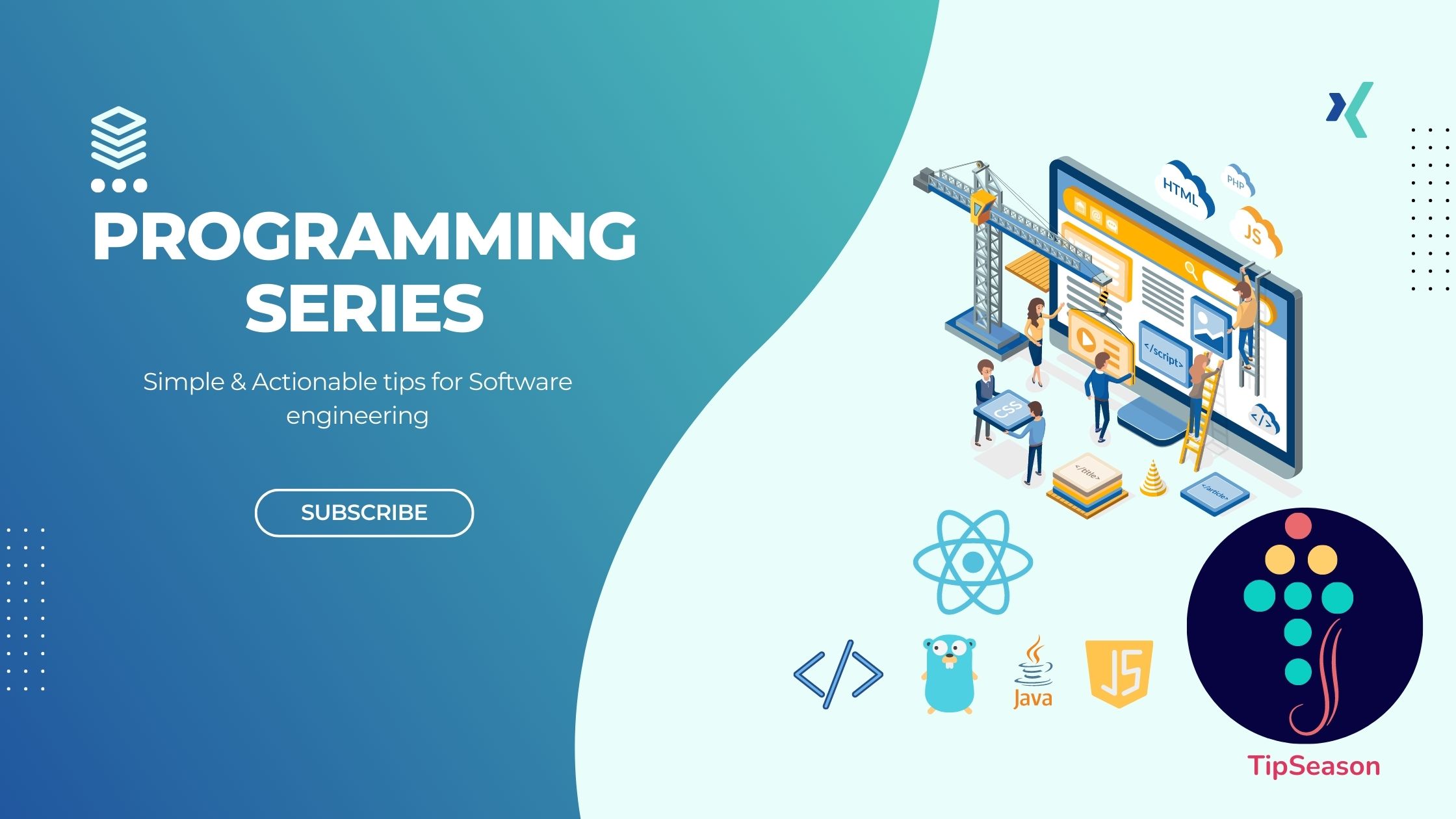
3 simple ways to get the first character of a string in Golang
In Golang, working with strings is a common task in many programming scenarios. Often, we need to extract or manipulate a certain character from a string, and the first character is one such example.
Getting the first character of a string may seem like a trivial task, but it can be done in multiple ways in Golang. In this blog post, we will explore three simple ways to accomplish this task using built-in functions and methods in the Go programming language.
By the end of this article, you will have a good understanding of these methods and be able to choose the one that best suits your needs. these methods in detail.
In case you missed it check out other Golang articles here
Golang read file line by line - 3 Simple ways
Golang get last element of slice / array / list - Simple and fast
Golang get map keys - 3 Simple ways
Golang enums implementation with examples
Golang sets implementation in 2 simple ways
Golang for loops: 5 basic ways with examples using range, arrays, maps iteration
Simple HTTP server with java socket programming
So, let’s dive in and explore the 3 simple ways to get the first character of a string in Golang.
1. Use the index position to access the string characters
One way to get the first character of a string in Golang is to use the index position of the first character, which is 0. We can access a character in a string by its index position using the square bracket notation.
To get the first character of a string, we can simply use the index position 0. For example, if we have a string “hello”, we can get the first character “h” by using “string[0]”.
Here’s an example code snippet:
1
2
3
4
5
6
7
8
9
10
11
package main
import "fmt"
func main() {
str := "This is a string from TipSeason.com"
fmt.Printf("%c", str[0])
}
Output:
$ go run example.go
T
In the above code snippet, we have declared a string “This is a string from TipSeason.com” and used the index position 0 to access the first character of the string. We have then converted the byte value to a string using the “string” keyword. Finally, we have printed the first character “T” using the “fmt.Printf” function.
This method is simple and straightforward, but we need to be careful to ensure that the string is not empty before accessing the first character to avoid runtime errors.
2. Use a slice expression on a string to get the section of the string that you want
Another way to get the first character of a string in Golang is to use a slice expression to extract a section of the string that we want. A slice expression is a way to extract a portion of a string by specifying a starting and ending index.
To get the first character of a string using a slice expression, we can specify the starting index as 0 and the ending index as 1. This will extract a section of the string containing only the first character.
Here’s an example code snippet:
1
2
3
4
5
6
7
8
9
10
11
package main
import "fmt"
func main() {
str := "This is a string from TipSeason.com"
fmt.Printf(str[0:1])
}
Output:
$ go run example.go
T
In the above code snippet, we have declared a string “This is a string from TipSeason.com” and used a slice expression to extract a section of the string containing only the first character. The slice expression [0:1] specifies the starting index as 0 and the ending index as 1, which will extract a section of the string containing only the first character. Finally, we have printed the first character “T” using the “fmt.Printf” function.
This method is also simple and easy to understand. It doesn’t require us to know the length of the string or the index position of the first character. However, it does require us to use a slice expression, which may not be familiar to some programmers who are new to Golang.
3. Use the string() function to convert a string to a slice of bytes.
The third way to get the first character of a string in Golang is to use the “string()” function to convert a string to a slice of bytes. Once we have the slice of bytes, we can access the first byte using the index position 0 and convert it back to a string using the “string()” function.
Here’s an example code snippet:
1
2
3
4
5
6
7
8
9
10
11
12
package main
import "fmt"
func main() {
str := "This is a string from TipSeason.com"
fmt.Printf("%c", string(str)[0])
}
Output:
$ go run example.go
T
Note that the first two methods work on ASCII characters, while the third method works on all characters.
In the above code snippet, we have declared a string “This is a string from TipSeason.com” and used the “string()” function to convert it to a slice of bytes. We have then accessed the first byte of the slice using the index position 0 and converted it back to a string using the “string()” function. We have then printed the first character “T” using the “fmt.Printf” function. This method is not straightforward, but the advantage is that first two methods work on ASCII characters. While the third method worls for all characters.
Conclusion
In conclusion, we have explored three simple ways to get the first character of a string in Golang. These methods include using the index position, using a slice expression, and using the “string()” function.
Each method has its advantages and disadvantages, and the best method to use will depend on the specific requirements of your programming scenario.
We hope this article has been informative and helpful in your Golang programming endeavors. If you enjoyed this article and want to learn more about Golang or programming in general, be sure to subscribe to our blog for more great content like this. Thank you for reading!
We hope you like this post. If you have any questions or suggestions or need any other additional info, please comment below.
We have started a coding community for most frequently used real world coding tips. You can join us here
TipSeason Discord channel
TipSeason Facebook Group
What do you want to learn next ? Drop a comment below!1600+ Midjourney prompts + 1500 AI Art prompt keywords, 25 categories, logo, tshirt, coloring page, characters , AI art prompts, Digital art https://t.co/X4zMgNepnk via @Etsy
— TipSeason⚡💡 (@thetipseason) April 11, 2023