Golang get last element of slice / array / list - Simple and fast
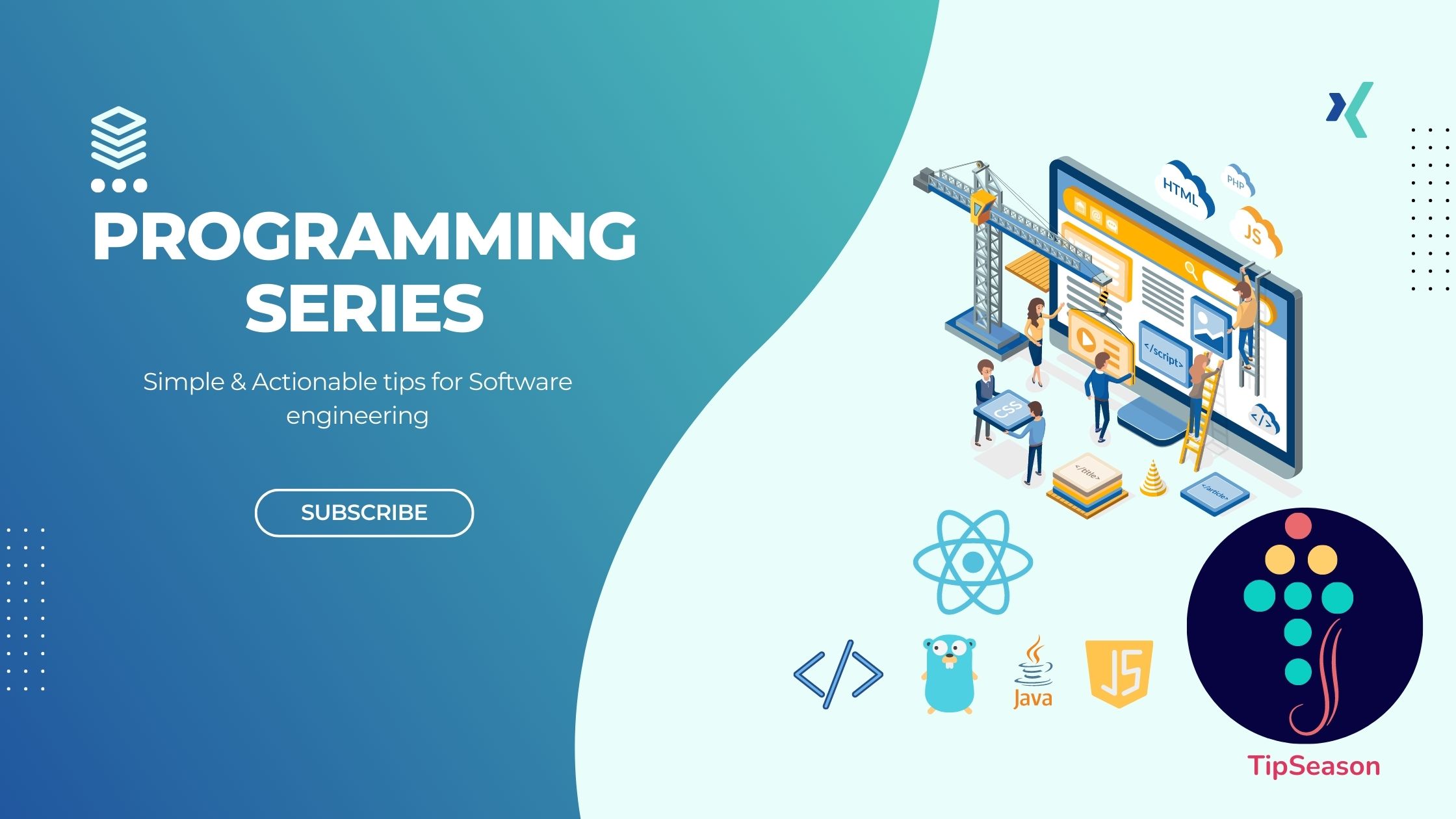
Simple ways to get the last element of a slice or an array or list
When working with production code in Golang, efficiently accessing the last element of a slice or an array or a list is a common requirement. In this blog post, we’ll explore simple and fast ways to achieve this task, ensuring both readability and performance in your Golang code.
In case you missed it check out other Golang articles here
3 simple ways to get the first character of a string in Golang
Golang read file line by line - 3 Simple ways
Golang enums implementation with examples
Golang sets implementation in 2 simple ways
Golang get map keys - 3 Simple ways
Golang for loops: 5 basic ways with examples using range, arrays, maps iteration
So, let’s dive in and explore the 3 simple ways to get the first character of a string in Golang.
1. Get last element in a slice or array in golang:
One straightforward approach to obtaining the last element of a Golang slice is by using indexing. In Go, slices are zero-indexed, and you can leverage this property to access the last element conveniently.
1
lastElement := mySlice[len(mySlice)-1]
Example:
1
2
3
4
5
6
7
8
9
10
11
package main
import "fmt"
func main() {
mySlice := []int{1, 2, 3, 4, 5}
lastElement := mySlice[len(mySlice)-1]
fmt.Println("Last Element:", lastElement)
}
Output:
1
Last Element: 5
2. Get last element in a list using Go:
The Back()
method is used to access the last element of a list in Golang.
It returns a pointer to the last element, which you can then use to access the Value field and type-assert it to the appropriate type.
Remember that this method is only available in Go 1.18+.
1
list.Back().Value
Example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
package main
import (
"container/list"
"fmt"
)
func main() {
// Sample slice of integers
numbers := []int{1, 2, 3, 4, 5}
// Convert slice to list
tempList := list.New()
for _, num := range numbers {
tempList.PushBack(num)
}
// Get the last element using the Back() method
lastElement := tempList.Back().Value.(int) // Note: Using Back() method
// Print the last element
fmt.Println("Last element:", lastElement) // Output: 5
}
Output:
1
Last element: 5
Conclusion
We have explored easy ways to get the last elemtn in a list or a slice in Go.
We hope this article has been informative and helpful in your Golang programming endeavors. If you enjoyed this article and want to learn more about Golang or programming in general, be sure to subscribe to our blog for more great content like this. Thank you for reading!
We hope you like this post. If you have any questions or suggestions or need any other additional info, please comment below.
We have started a coding community for most frequently used real world coding tips. You can join us here
TipSeason Discord channel
TipSeason Facebook Group
What do you want to learn next ? Drop a comment below!1600+ Midjourney prompts + 1500 AI Art prompt keywords, 25 categories, logo, tshirt, coloring page, characters , AI art prompts, Digital art https://t.co/X4zMgNepnk via @Etsy
— TipSeason⚡💡 (@thetipseason) April 11, 2023